Definition of API
API which stands for Application Programming Interface, in short is just a glorified data access layer. It also enables two software components to communicate with each other using a set of protocols. For eg: If you want to access the messages on your Instagram application; It is updated via the internal API of the Instagram.
Just like we humans communicate each other using our own language, computers talk with servers with APIs. There are many type of APIs like RPC, GRPC, Websocket, REST, etc. Here I am going to explain REST API. One device sends a request to a server, the server validates the request & gives a appropriate response then the received response is then shown in the UI.
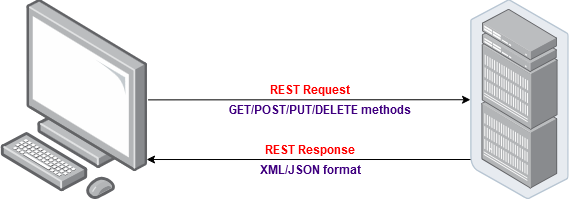
If you are still having hard time understanding API, then imagine that you’re at a restaurant. You obviously don’t go to their kitchen and make food yourself to eat; I doubt they will even let you enter in their kitchen. So, you will tell the waiter what you want from the menu, so that your order is delivered to kitchen. Then, the waiter brings the food back to you once it is ready. In this example:
- You are like a computer or a program.
- The waiter is the API.
- The kitchen is the server where the actual work (cooking or processing data) happens.
The waiter is the API, it takes your request (your food order), tells the kitchen (the server) what to do, and then brings the result (your food) back to you.
Why is validation required?
Imagine a world where anyone could access anyone else’s private messages—chaotic, right? All your personal data, messages, and sensitive information would be exposed for anyone to see and misuse. To avoid this chaos, validation is essential in every system, especially when dealing with APIs.
Validation ensures that only authorized users and programs can access specific data or perform certain actions. Every request sent to a server through an API goes through a series of validations to ensure it is:
- Authorized: Does this person or application have permission to perform this action?
- Authentic: Is the person or application making the request who they claim to be?
Token-Based Validation: A Simple Example
One common way to validate API requests is through token-based validation. Here’s how it works:
- When you log in to an application, the system validates your credentials (e.g., username and password).
- If your credentials are correct, the server generates a unique token just for you. Think of it like a key to your personal vault of data.
- This token must be sent along with every API request you make to the server.
- The server checks if the token is valid and associated with the correct user. If the token is missing or invalid, the request is rejected as unauthenticated.
By requiring a token for every request, the server ensures that only authorized users can access or modify specific data, keeping your information safe and private.
Validation using sanctum
When working with Laravel, one of the simplest and most popular ways to validate API requests is by using Sanctum.
Sanctum provides a lightweight authentication system for Single Page Applications (SPAs), mobile applications, and simple token-based APIs. It’s an easy-to-implement solution that comes with built-in support for issuing and validating tokens.
Here’s how Sanctum works:
- Token Generation:
- Once a user logs in, Sanctum generates a token for that user.
- The token is stored securely on the client-side (e.g., in cookies or local storage).
- Making Requests:
- For every API request, the client sends the token along with the request (usually in the
Authorization
header).
- For every API request, the client sends the token along with the request (usually in the
- Validation Process:
- Sanctum verifies the token against its records to ensure it’s valid and hasn’t expired.
- If the token matches and the user has the necessary permissions, the request is processed. Otherwise, it’s rejected.
Code sample for Token based authentication
api.php
Route::post('/name', function () {
dd('Diwash is don');
})->middleware('auth:sanctum');
Route::post('/login', [\App\Http\Controllers\Api\AuthController::class, 'login']);
AuthController.php
class AuthController extends Controller
{
use APIResponses;
public function login(LoginUserRequest $request)
{
$request->validated($request->all());
if (!Auth::attempt($request->only('email', 'password'))) {
return $this->error('Invalid credentials', 401);
}
$user = User::firstWhere('email', $request->email);
return $this->ok(
'Authenticated',
[
'token' => $user->createToken('API token for ' . $user->email)->plainTextToken
]
);
}
}
User.php
class User extends Authenticatable
{
/** @use HasFactory<\Database\Factories\UserFactory> */
use HasApiTokens, HasFactory, Notifiable;
/**
* The attributes that are mass assignable.
*
* @var list<string>
*/
protected $fillable = [
'name',
'email',
'password',
];
/**
* The attributes that should be hidden for serialization.
*
* @var list<string>
*/
protected $hidden = [
'password',
'remember_token',
];
/**
* Get the attributes that should be cast.
*
* @return array<string, string>
*/
protected function casts(): array
{
return [
'email_verified_at' => 'datetime',
'password' => 'hashed',
];
}
}
APIResponses.php
trait APIResponses
{
protected function ok($message, $data): JsonResponse
{
return $this->successResponse($message, $data, 200);
}
protected function error($message): JsonResponse
{
return $this->errorResponse($message, 400);
}
protected function successResponse($message, $data, $statusCode = 200): JsonResponse
{
return response()->json([
'message' => $message,
'data' => $data,
'status' => $statusCode
], $statusCode);
}
protected function errorResponse($message, $statusCode = 400): JsonResponse
{
return response()->json([
'message' => $message,
'status' => $statusCode
], $statusCode);
}
}
Postman
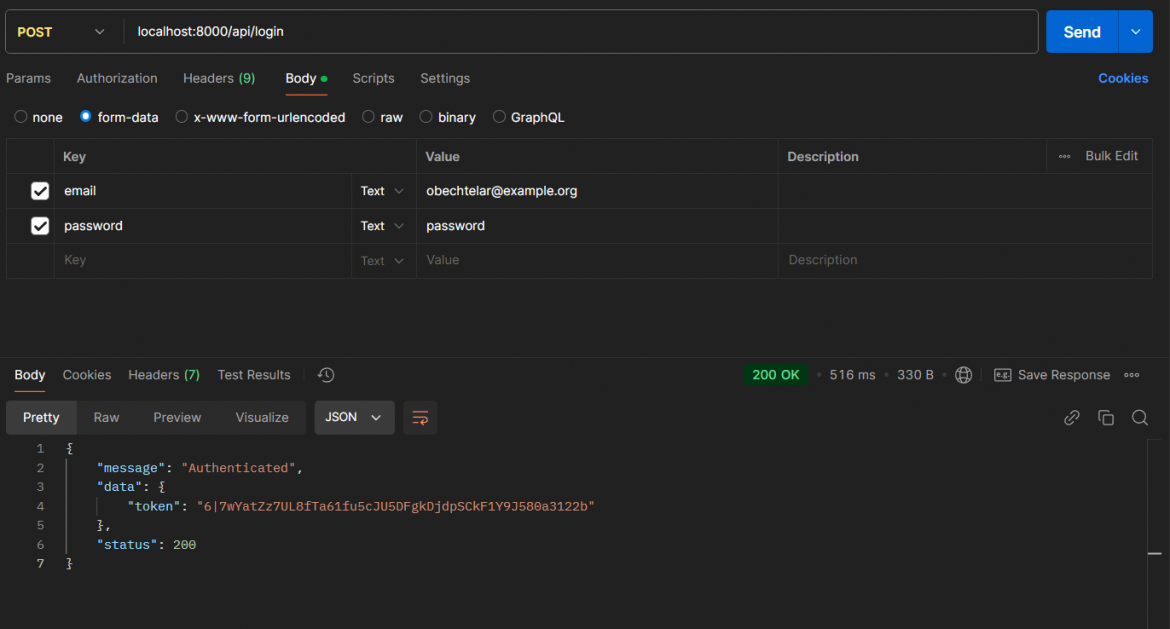
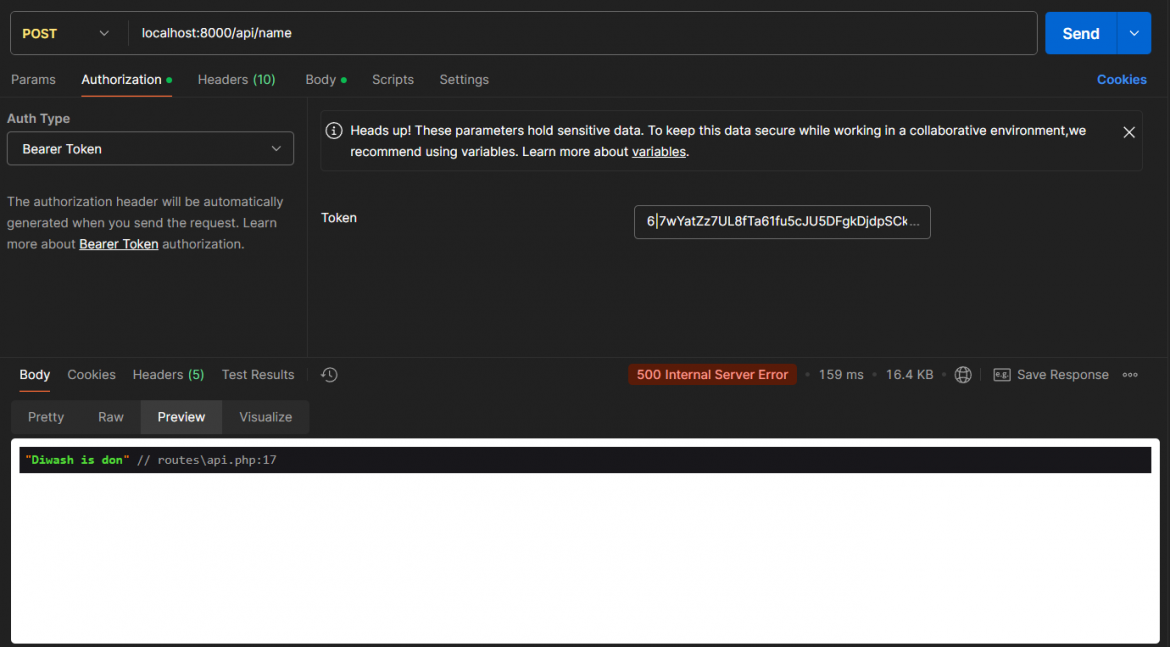
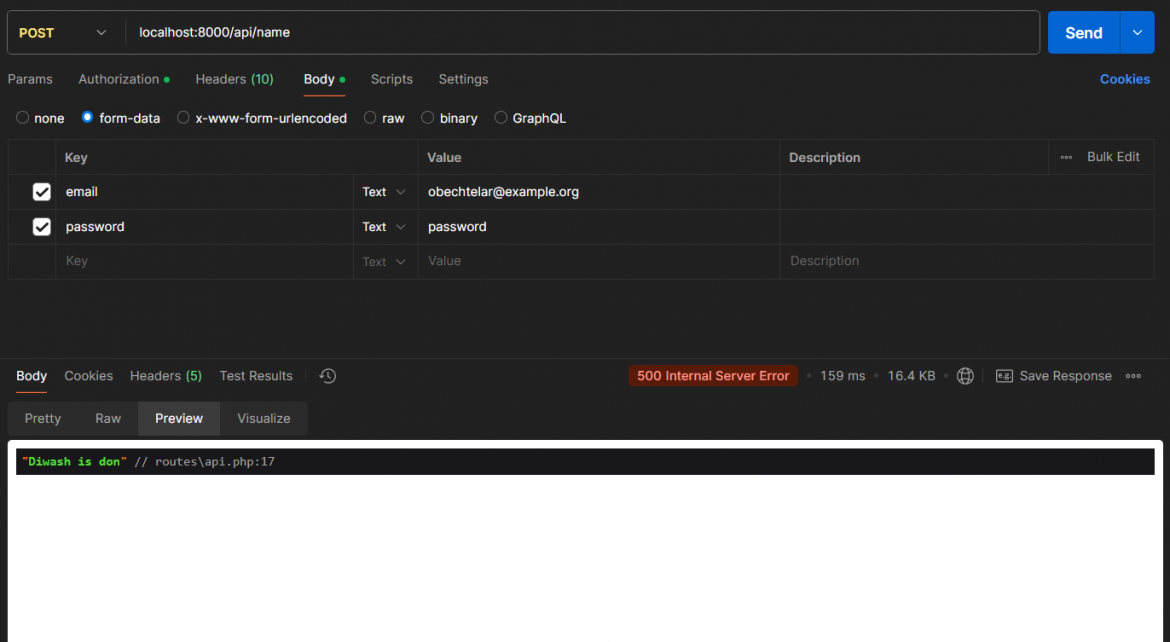
Why Choose Sanctum for API Validation?
Sanctum is the default choice in Laravel for API validation because:
- Ease of Use: Sanctum is straightforward to set up and integrates seamlessly with Laravel.
- Token Management: It provides out-of-the-box functionality for issuing, revoking, and managing tokens.
- Support for SPAs and APIs: Sanctum is versatile enough to handle authentication for both Single Page Applications and traditional APIs.
- Security: Sanctum ensures that every token is tied to a specific user and can be scoped to limit access to certain actions or resources.